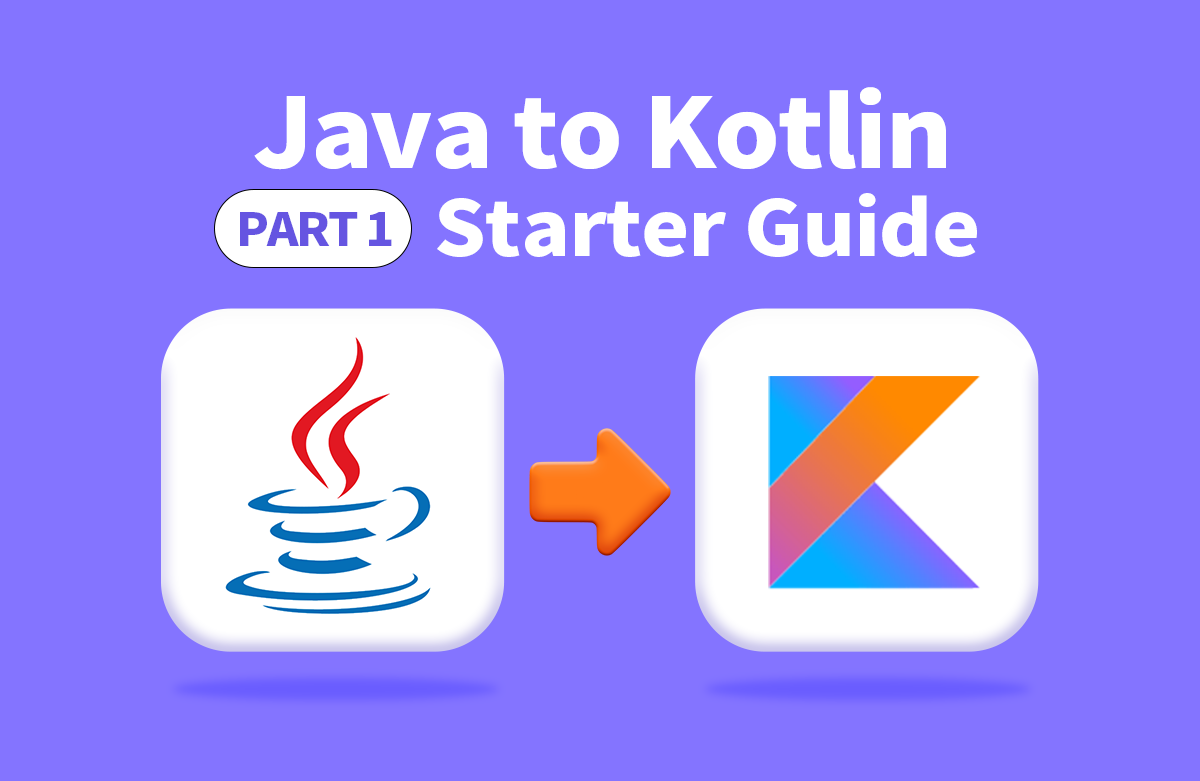
1. 필터와 맵
- filter
특정 조건을 만족하는 데이터만 List에 저장
val apples = fruits.filter { fruit -> fruit.name == "사과" }
- filterIndexed
filter에서 인덱스가 함께 필요할 경우
val apples = fruits.filterIndexed { idx, fruit ->
println(idx)
fruit.name == "사과"
}
- map
필터링 후 특정 데이터만 저장하고 싶은 경우
val applePrices = fruits.filter { fruit -> fruit.name == "사과" }
.map { fruit -> fruit.currentPrice }
- mapIndexed
map에서 인덱스가 함께 필요한 경우
val applePrices = fruits.filter { fruit -> fruit.name == "사과" }
.mapIndexed { idx, fruit ->
println(idx)
fruit.currentPrice
}
- mapNotNull
map에서 null이 아닌 값만 저장하고 싶은 경우
val applePrices = fruits.filter { fruit -> fruit.name == "사과" }
.mapNotNull { fruit -> fruit.nullOrValue() }
2. 다양한 컬렉션 처리 기능
- all
조건을 모두 만족하면 true, 그렇지 않다면 false
val isAllApple = fruits.all { fruit -> fruit.name == "사과" }
- none
조건을 모두 불만족하면 true, 그렇지 않다면 false
val isAllApple = fruits.none { fruit -> fruit.name == "사과" }
- any
조건을 하나라도 만족하면 true, 그렇지 않다면 false
val isPrice = fruits.any { fruit -> fruit.price >= 10_000 }
- count
총 개수
val count = fruits.count()
- sortedBy
오름차순 정렬
val sortFruits = fruits.sortedBy { fruit -> fruit.price }
- soredByDescending
내림차순 정렬
val sortFruits = fruits.sortedByDescending { fruit -> fruit.price }
- distinct
변형된 값을 기준으로 중복을 제거
val fruitsNames = fruits.distinctBy { fruit -> fruit.name }
.map {fruit -> fruit.name }
- first
첫번 째 값을 가져온다 (null일 경우 예외 발생)
fruits.first()
- firstOrNull
첫번 째 값을 가져온다. 값이 없을 경우 null을 가져온다
fruits.firstOrNull()
- last
마지막 값을 가져온다 (null일 경우 예외 발생)
fruits.last()
- lastOrNull
마지막 값을 가져온다. 값이 없을 경우 null을 가져온다
fruits.lastOrNull()
3. List to Map
- groupBy
조건 값을 기준으로 묶어서 map으로 추출하고 싶을 때
ex) 과일 이름을 key값으로 List를 저장하고 싶을 때
val fruitsMap: Map<String, List<Fruit>> =
fruits.groupBy { fruit -> fruit.name }
/* 응용도 가능 */
val fruitsMap: Map<String, List<Fruit>> =
fruits.groupBy { fruit -> fruit.name }
.filter { (key, value) -> key == "사과" }
List에 특정 값만 묶어서 map으로 추출하고 싶을 때
ex) 과일 이름을 Key값으로 가격만 List로 저장하고 싶을 때
val fruitsMap: Map<String, List<Long>> =
fruits.groupBy({ fruit -> fruit.name }, { fruit -> fruit.price })
- associateBy
중복되지 않는 키를 가지고 Map을 만들고 싶을 때
ex) 과일 ID를 Key값으로 과일 객체를 저장하고 싶을 때
val fruitMap: Map<Long, Fruit> =
fruits.associateBy { fruit -> fruit.id }
객체가 아닌 특정 값을 value로 저장하고 싶을 때
ex) 과일 ID를 Key값으로 가격 값을 저장하고 싶을 때
val fruitMap: Map<Long, Long> =
fruits.associateBy({ fruit -> fruit.id }, { fruit -> fruit.price })
4. 중첩된 컬렉션 처리
- flatMap
List안에 중첩된 List들이 있는 자료 구조일 경우 조건에 만족하는 데이터만 단일 List로 변경해준다
ex) 출고가와 현재가가 동일한 과일을 단일 List로 저장하고 싶을 때
val fruitsInList: List<List<FruitDto>> = listOf(
listOf(
FruitDto(1L, "사과", 1_000, 1_500),
FruitDto(2L, "사과", 1_200, 1_500),
FruitDto(3L, "사과", 1_500, 1_500),
),
listOf(
FruitDto(4L, "바나나", 1_000, 1_500),
FruitDto(5L, "바나나", 1_200, 1_500),
FruitDto(6L, "바나나", 1_500, 1_500),
),
listOf(
FruitDto(7L, "수박", 1_000, 1_500),
)
)
var samePriceFruits = fruitsInList.flatMap { list ->
list.filter { fruit -> fruit.factoryPrice == fruit.currentPrice }
}
List 안에 또 List를 필터링하는 람다가 있기 때문에 가독성이 떨어진다
아래와 같이 리팩토링이 가능하다
data class FruitDto(
val id: Long,
val name: String,
val factoryPrice: Long,
val currentPrice: Long,
) {
/* custom getter 추가 */
val isSamePrice: Boolean
get() = this.factoryPrice == this.currentPrice
}
/* 확장 함수 추가 */
val List<FruitDto>.samePriceFilter: List<FruitDto>
get() = this.filter(FruitDto::isSamePrice)
/*var samePriceFruits = fruitsInList.flatMap { list ->
list.filter { fruit -> fruit.factoryPrice == fruit.currentPrice }
}*/
var samePriceFruits = fruitsInList.flatMap { list -> list.samePriceFilter }
- flatten
중첩되어있는 컬렉션을 중첩 해제 시킬 때 사용
ex) List<List<Fruit>> -> List<Fruit>로 변경
fruitsInList.flatten()
인프런 강의
[자바 개발자를 위한 코틀린 입문] part1 - 최태현 강사님
https://www.inflearn.com/course/java-to-kotlin/dashboard
'개발 지식 > 자바 개발자를 위한 코틀린 입문' 카테고리의 다른 글
자바 개발자를 위한 코틀린 입문 - scope function (1) | 2023.08.11 |
---|---|
자바 개발자를 위한 코틀린 입문 - 다양한 함수 (0) | 2023.08.10 |
자바 개발자를 위한 코틀린 입문 - Sealed Class (0) | 2023.08.09 |
자바 개발자를 위한 코틀린 입문 - 상속 방법과 주의점 (0) | 2023.08.09 |
자바 개발자를 위한 코틀린 입문 - 동일성/동등성 비교 방법 (0) | 2023.08.07 |